As a part of our flutter tutorial series, we’re going to learn how to implement dropdown button with flutter.
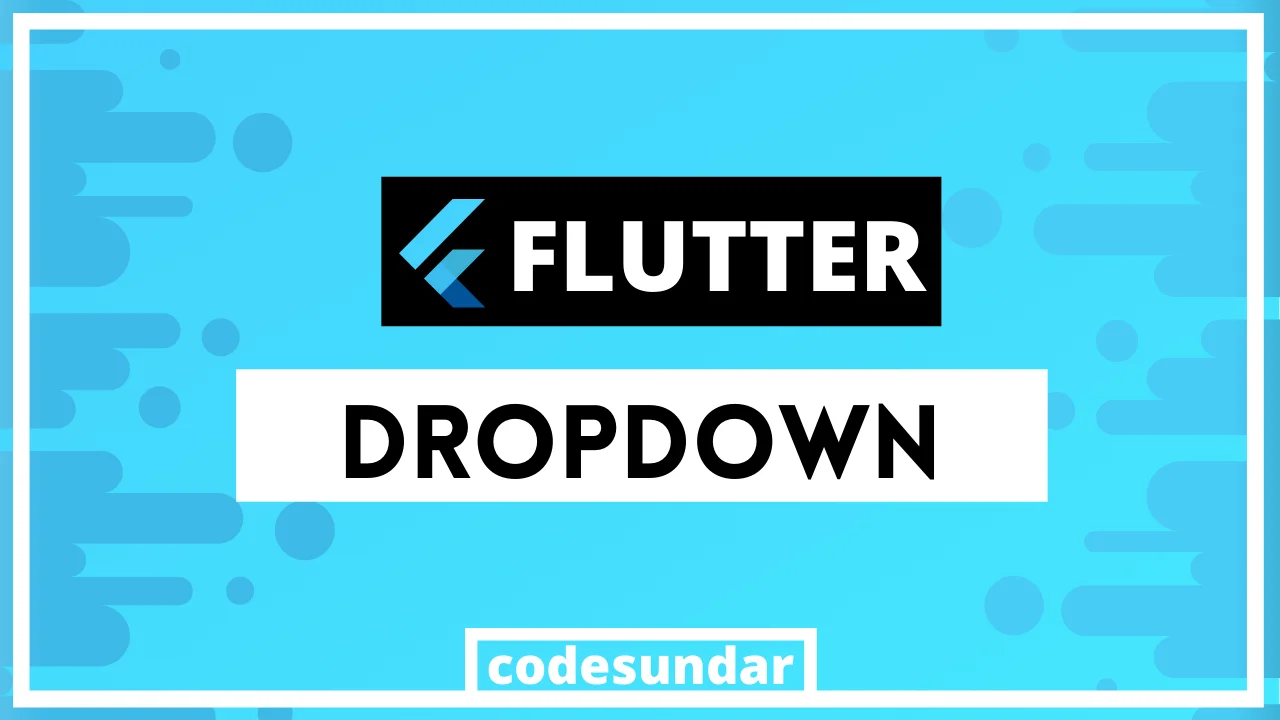
Flutter Dropdown Button Example Source code
class _HomePageState extends State {
String _selectedItem = 'Sun';
List _options = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("DropDown Example"),
),
body: Container(
width: double.infinity,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text("Selected Day: $_selectedItem"),
DropdownButton(
value: _selectedItem,
items: _options
.map(
(day) => DropdownMenuItem(
child: Text(day),
value: day,
),
)
.toList(),
onChanged: (value) {
setState(() {
_selectedItem = value;
});
},
),
]),
),
);
}
}
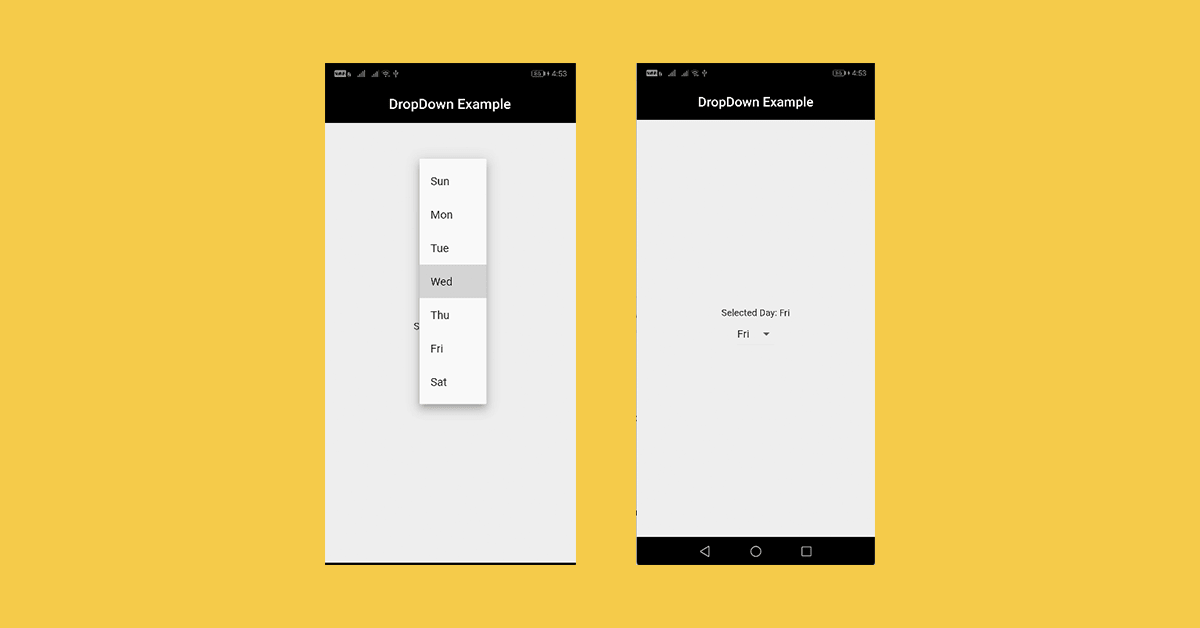
Additional Resources