As a part of flutter tutorial series, we’re learn how to access user geolocation in flutter with example. This example we’re going to use location Package (http://pub.dev/packages/location)
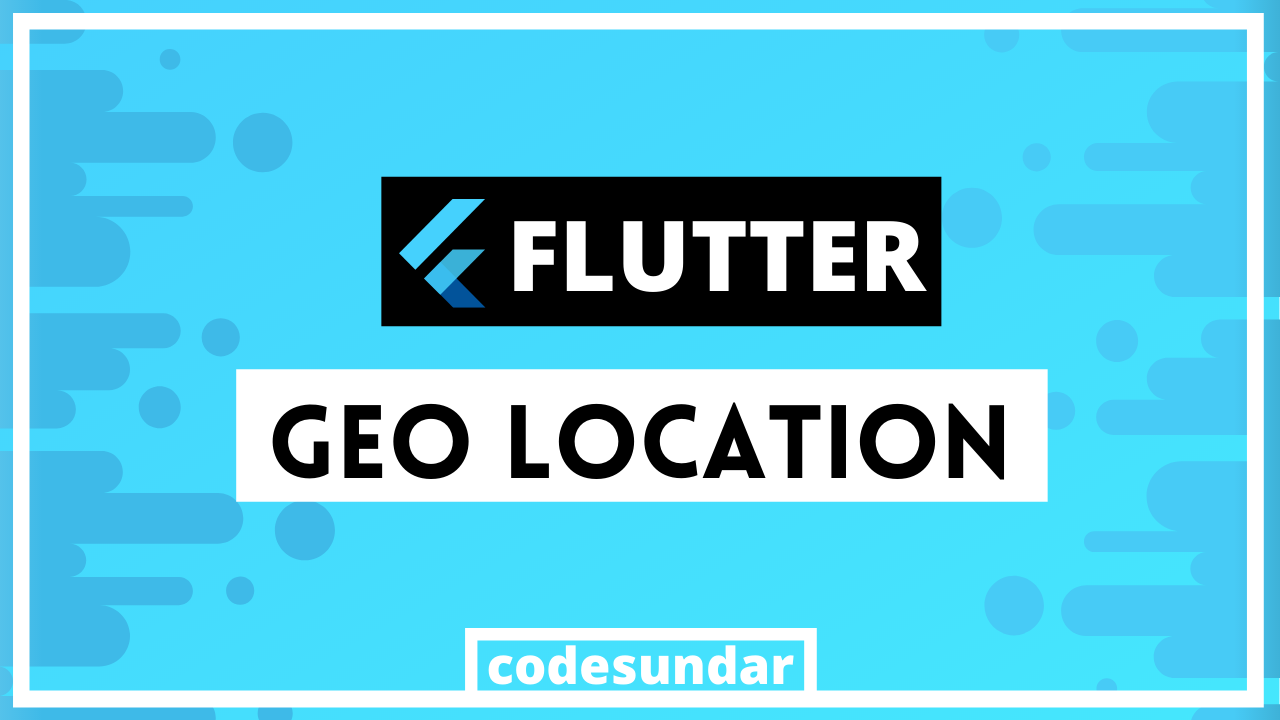
Usage:
- Geolocation used in many application such as On-demand taxi booking app, delivery app, tracking app and more.
What we’ll learn?
- Access current location of device using flutter?
- Track geolocation change in flutter
- Working with Location Permission
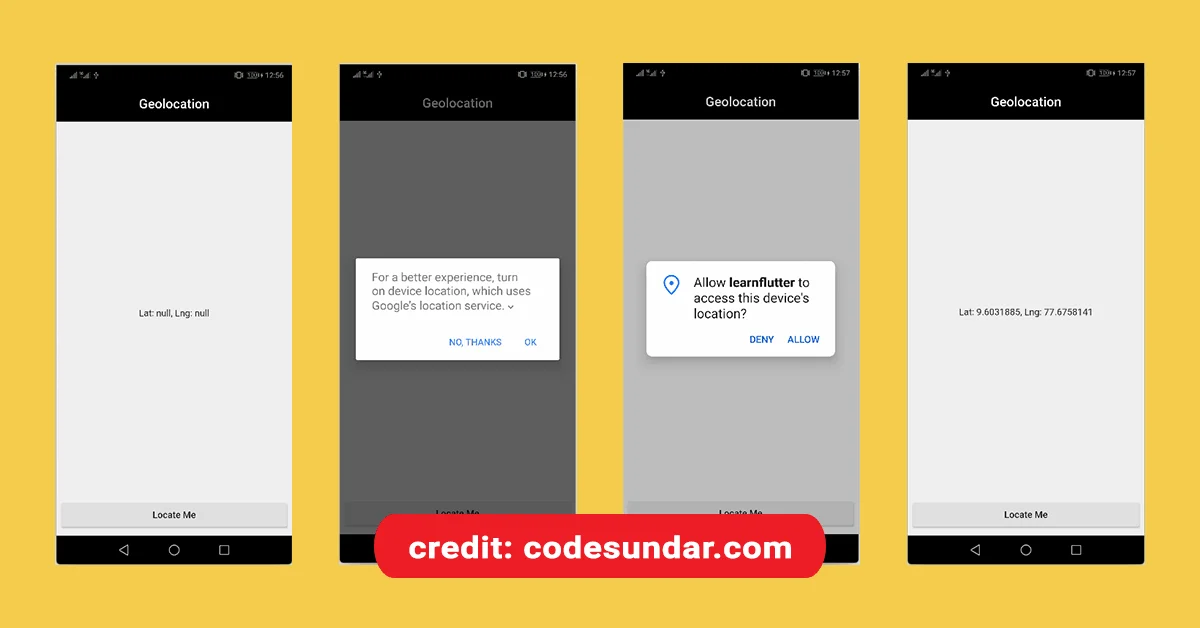
Getting Started
To start, first we need to create a new flutter project or you can use any existing flutter project.
flutter create learnflutter
cd learnflutter
Then open pubspec.yaml
file and add location package.
flutter pub get
This will download all required packages and configure it automatically for android. if you’re using other platform such as ios or web please refer http://pub.dev/packages/location for configuration.
Access User Location using Flutter Example 1
class _HomePageState extends State<HomePage> {
double lat;
double lng;
Location location = new Location();
bool _serviceEnabled;
PermissionStatus _permissionGranted;
_locateMe() async {
_serviceEnabled = await location.serviceEnabled();
if (!_serviceEnabled) {
_serviceEnabled = await location.requestService();
if (!_serviceEnabled) {
return;
}
}
_permissionGranted = await location.hasPermission();
if (_permissionGranted == PermissionStatus.denied) {
_permissionGranted = await location.requestPermission();
if (_permissionGranted != PermissionStatus.granted) {
return;
}
}
await location.getLocation().then((res) {
setState(() {
lat = res.latitude;
lng = res.longitude;
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Geolocation"),
),
body: Container(
width: double.infinity,
padding: EdgeInsets.all(8.0),
child: Column(
children: [
Expanded(
child: Center(
child: Text("Lat: $lat, Lng: $lng"),
),
),
Container(
width: double.infinity,
child: RaisedButton(
child: Text("Locate Me"),
onPressed: () => _locateMe(),
),
),
],
),
),
);
}
}
Code Explanation:
- Here, we created a RaisedButton and Text widgets are aligned in column and expanded widget.
- We created variable called
lat
,lng
to store user location. we also created few variable for service status and permission - When user click button, we’re calling
locateMe()
- Inside
locateMe()
, first we’re checking for location service status, if it’s not true, we’re requesting permission to activate it. - Once location service is activated, we’re requesting location permission for our app. once permission is given, now we can access device location.
Track User Location in Flutter – Example 2
import 'package:flutter/material.dart';
import 'package:location/location.dart';
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
double lat;
double lng;
Location location = new Location();
bool _serviceEnabled;
PermissionStatus _permissionGranted;
_locateMe() async {
_serviceEnabled = await location.serviceEnabled();
if (!_serviceEnabled) {
_serviceEnabled = await location.requestService();
if (!_serviceEnabled) {
return;
}
}
_permissionGranted = await location.hasPermission();
if (_permissionGranted == PermissionStatus.denied) {
_permissionGranted = await location.requestPermission();
if (_permissionGranted != PermissionStatus.granted) {
return;
}
}
// Track user Movements
location.onLocationChanged.listen((res) {
setState(() {
lat = res.latitude;
lng = res.longitude;
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Geolocation"),
),
body: Container(
width: double.infinity,
padding: EdgeInsets.all(8.0),
child: Column(
children: [
Expanded(
child: Center(
child: Text("Lat: $lat, Lng: $lng"),
),
),
Container(
width: double.infinity,
child: RaisedButton(
child: Text("Locate Me"),
onPressed: () => _locateMe(),
),
),
],
),
),
);
}
}
Code Explanation:
- Design and concepts are same as above example.
- Additionally, we used
location.onLocationChanged.listen()
to listen location changes and update the values.