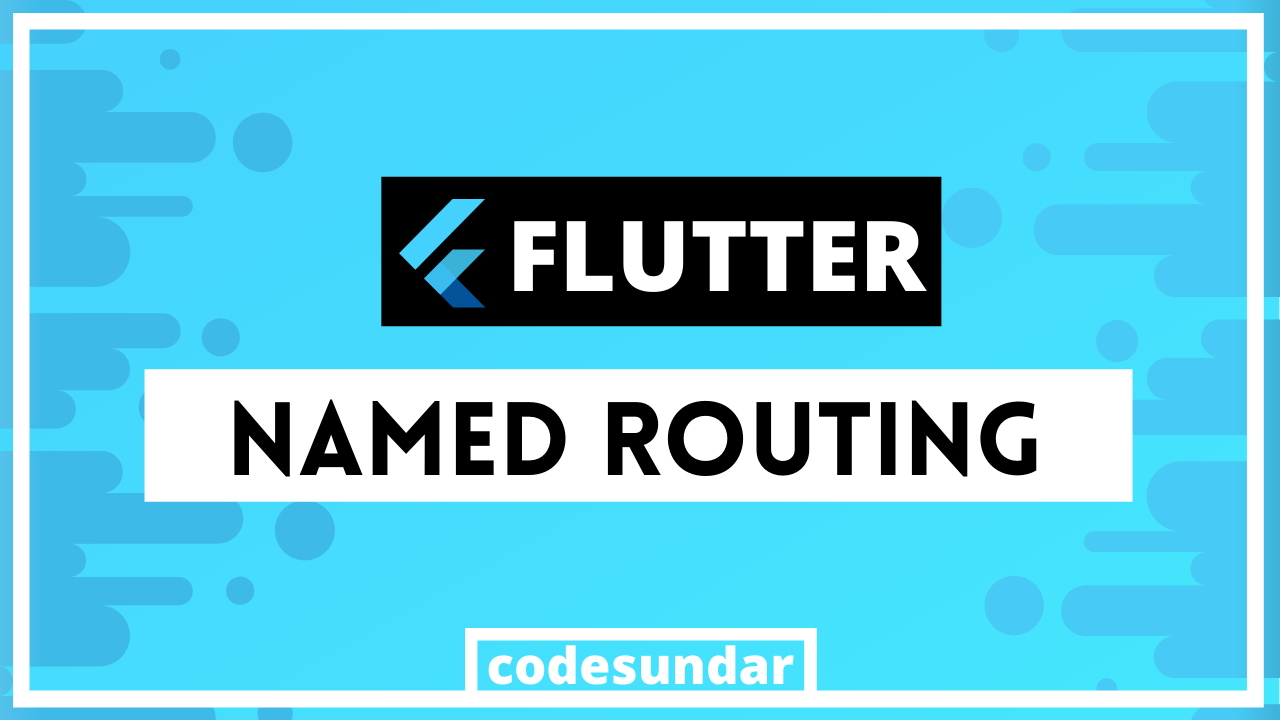
In our flutter tutorial series, I’m going to write a flutter named routing tutorial. In the last article, we have seen how to use simple routing with flutter.
Getting started
Let’s create a new project
flutter create navigate
<span class="hljs-built_in">cd</span> navigate
then, we need to create the required pages called first.dart
, second.dart
, and third.dart
inside pages folder.
lib/pages/first.dart
<span class="hljs-keyword">import</span> <span class="hljs-symbol">'package</span>:flutter/material.dart';
<span class="hljs-class"><span class="hljs-keyword">class</span> <span class="hljs-title">FirstPage</span> <span class="hljs-keyword">extends</span> <span class="hljs-title">StatelessWidget</span> </span>{
<span class="hljs-meta">@override</span>
<span class="hljs-type">Widget</span> build(<span class="hljs-type">BuildContext</span> context) {
<span class="hljs-comment">// <span class="hljs-doctag">TODO:</span> implement build</span>
<span class="hljs-keyword">return</span> <span class="hljs-type">Scaffold</span>(
appBar: <span class="hljs-type">AppBar</span>(title: <span class="hljs-type">Text</span>(<span class="hljs-string">"First Page"</span>)),
body: <span class="hljs-type">Center</span>(
child: <span class="hljs-type">Column</span>(children: <<span class="hljs-type">Widget</span>>[
<span class="hljs-type">Text</span>(<span class="hljs-string">"First Page"</span>),
<span class="hljs-type">RaisedButton</span>(child: <span class="hljs-type">Text</span>(<span class="hljs-string">"Goto Page 2"</span>), onPressed: () {
<span class="hljs-comment">// navigation code here</span>
})
]),
),
);
}
}
Explanation:
- We have created a single page/screen with Raisedbutton with center alignment
Note: you can create other pages with different class names like SecondPage and ThirdPage
After creating those pages, we need to declare our routes in MaterialApp() on main.dart
MaterialApp(
<span class="hljs-name">routes</span>: {
'/': (<span class="hljs-name">context</span>) => FirstPage(),
'/second': (<span class="hljs-name">context</span>) => SecondPage(),
'/third': (<span class="hljs-name">context</span>) => ThirdPage(),
},
)<span class="hljs-comment">;</span>
How to navigate using named routes?
You can use Navigator.pushNamed() for moving from one page to another
<span class="hljs-selector-tag">Navigator</span><span class="hljs-selector-class">.pushNamed</span>(context, <span class="hljs-string">'routeName'</span>);
<span class="hljs-selector-tag">Navigator</span><span class="hljs-selector-class">.pushNamed</span>(context, <span class="hljs-string">'/second'</span>);
now, we can use this syntax to update our first.dart
, second.dart
and third.dart
<span class="hljs-keyword">import</span> <span class="hljs-symbol">'package</span>:flutter/material.dart';
<span class="hljs-class"><span class="hljs-keyword">class</span> <span class="hljs-title">FirstPage</span> <span class="hljs-keyword">extends</span> <span class="hljs-title">StatelessWidget</span> </span>{
<span class="hljs-meta">@override</span>
<span class="hljs-type">Widget</span> build(<span class="hljs-type">BuildContext</span> context) {
<span class="hljs-comment">// <span class="hljs-doctag">TODO:</span> implement build</span>
<span class="hljs-keyword">return</span> <span class="hljs-type">Scaffold</span>(
appBar: <span class="hljs-type">AppBar</span>(title: <span class="hljs-type">Text</span>(<span class="hljs-string">"First Page"</span>)),
body: <span class="hljs-type">Center</span>(
child: <span class="hljs-type">Column</span>(
mainAxisAlignment: <span class="hljs-type">MainAxisAlignment</span>.center,
children: <<span class="hljs-type">Widget</span>>[
<span class="hljs-type">Text</span>(<span class="hljs-string">"First Page"</span>),
<span class="hljs-type">RaisedButton</span>(
child: <span class="hljs-type">Text</span>(<span class="hljs-string">"Goto Page 2"</span>),
onPressed: () => <span class="hljs-type">Navigator</span>.pushNamed(context, '/second'))
]),
),
);
}
}

Flutter Named Routing Tutorial: Passing data between pages
We all know, moving from one page to another page is not enough without passing data. This example, we’re going build a product catalog project for learning pass data between two pages. To do that, first, we need to create a new modal class for our product information.
<span class="hljs-class"><span class="hljs-keyword">class</span> <span class="hljs-title">Product</span></span>{
<span class="hljs-keyword">final</span> <span class="hljs-built_in">String</span> name;
<span class="hljs-keyword">final</span> <span class="hljs-built_in">double</span> price;
<span class="hljs-keyword">final</span> <span class="hljs-built_in">String</span> img;
Product({<span class="hljs-keyword">this</span>.name, <span class="hljs-keyword">this</span>.price, <span class="hljs-keyword">this</span>.img});
}
Then We have to create our Product List on our first page ( first.dart
) for displaying a product list.
List<Product> _products = [
Product(<span class="hljs-string">name:</span> <span class="hljs-string">"iPhone X"</span>, <span class="hljs-string">price:</span> <span class="hljs-number">60000</span>, <span class="hljs-string">img:</span> <span class="hljs-string">"http://rukminim1.flixcart.com/image/416/416/j9d3bm80/mobile/k/x/a/apple-iphone-x-mqa82hn-a-original-imaeyysgmypxmazk.jpeg?q=70"</span>),
Product(<span class="hljs-string">name:</span> <span class="hljs-string">"Samsung Galaxy A10"</span>, <span class="hljs-string">price:</span> <span class="hljs-number">19000</span>, <span class="hljs-string">img:</span> <span class="hljs-string">"http://rukminim1.flixcart.com/image/416/416/jt8yxe80/mobile/g/v/x/samsung-galaxy-a10-sm-a105fzbgins-original-imafenbrg4zt5xye.jpeg?q=70"</span>),
Product(<span class="hljs-string">name:</span> <span class="hljs-string">"Honor 8C "</span>, <span class="hljs-string">price:</span> <span class="hljs-number">13000</span>, <span class="hljs-string">img:</span> <span class="hljs-string">"http://rukminim1.flixcart.com/image/416/416/jq5iky80/mobile/h/z/n/honor-8c-bkk-al10-original-imafc7hyyxjpv6ew.jpeg?q=70"</span>),
];
Image Credit: flipkart.com
Using this list of data, we can create a list with ListView.builder()
<span class="hljs-selector-tag">ListView</span><span class="hljs-selector-class">.builder</span>(
<span class="hljs-attribute">itemCount</span>: _products.length,
<span class="hljs-attribute">itemBuilder</span>: (context, index){
<span class="hljs-selector-tag">return</span> <span class="hljs-selector-tag">ListTile</span>(
<span class="hljs-attribute">title</span>: Text(_products[index].name),
<span class="hljs-attribute">onTap</span>: (){
<span class="hljs-comment">// code here</span>
},
);
},
)
when a single product clicked, we need to pass our data to the next page using Navigator.pushNamed()
, where it takes the 3rd parameter for arguments. Here we have passed Product with every tap
Navigator.pushNamed(
context,
'/<span class="hljs-built_in">product</span>-<span class="hljs-built_in">info</span>',
argumen<span class="hljs-symbol">ts:</span> <span class="hljs-built_in">Product</span>(<span class="hljs-built_in">na</span><span class="hljs-symbol">me:</span> _products[<span class="hljs-built_in">index</span>].name, pri<span class="hljs-symbol">ce:</span> _products[<span class="hljs-built_in">index</span>].<span class="hljs-built_in">price</span>, i<span class="hljs-symbol">mg:</span> _products[<span class="hljs-built_in">index</span>].img),
);
Let’s create new page called product-detail.dart
& receive data using ModalRoute.of(context).settings.arguments
& Store into _productInfo
<span class="hljs-keyword">final</span> <span class="hljs-built_in">Product</span> _productInfo = ModalRoute.of(context).settings.arguments;