As a part of the flutter tutorial series, we’re going to learn row and column with example. In flutter development row and column are playing a very important role in UI design. let’s explore it
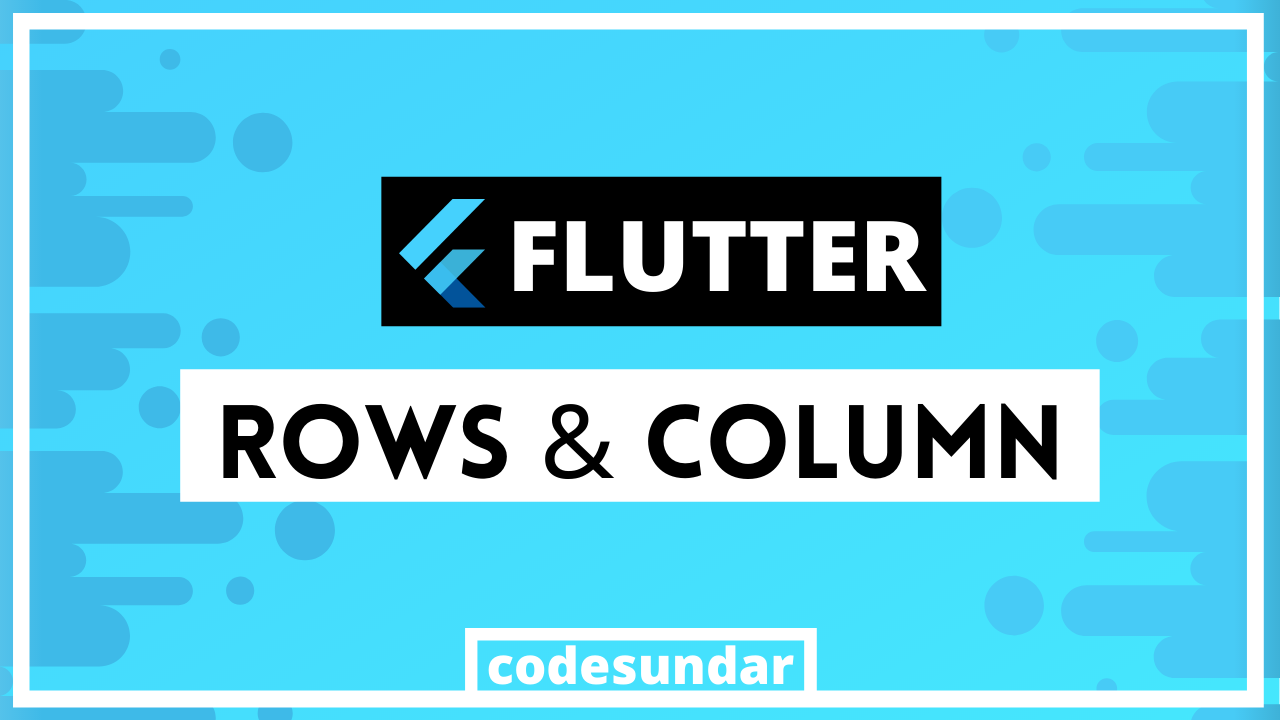
Row & Column comparison
Column | Row |
MultiChild Widget | MultiChild Widget |
Align all Children Widgets in a vertical direction | Align all children Widgets in a horizontal direction |
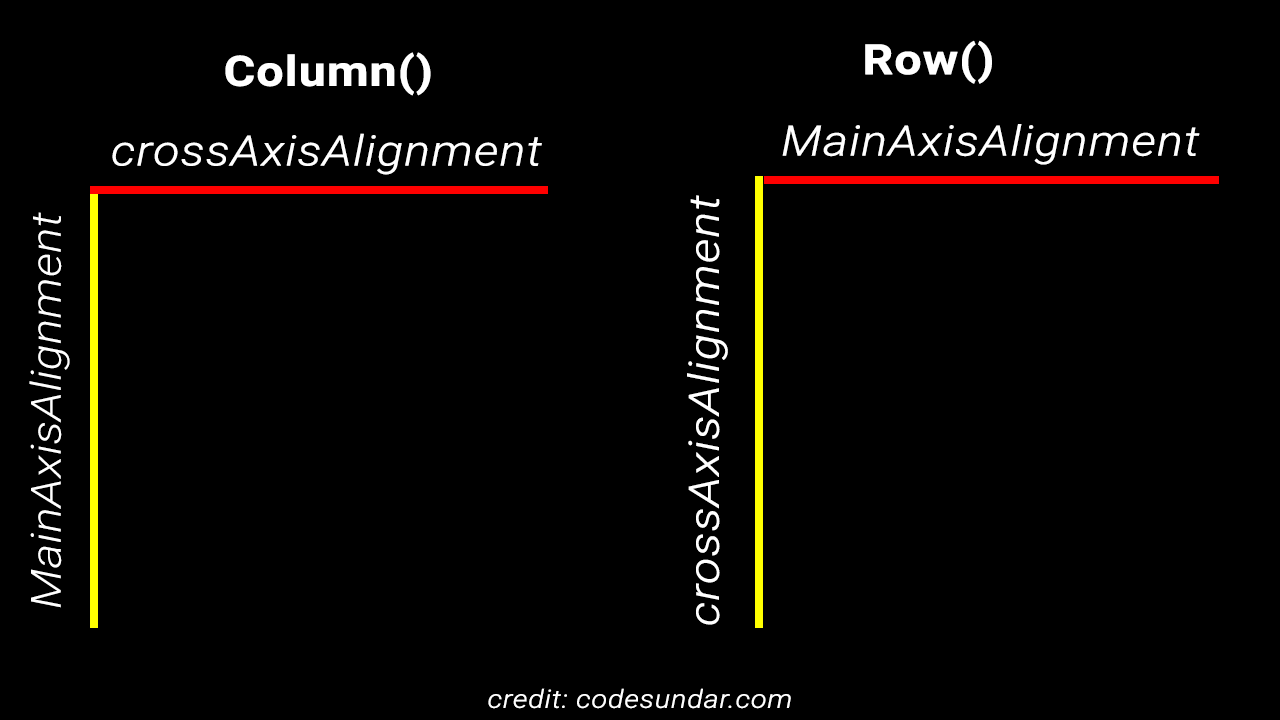
Exploring Column Widget
As said before, the column widget aligns the children’s widget vertical direction. To change the UI, we need to play with CrossAxisAligment & MainAxisAlignment. In the Column widget, MainAxisAligment will be in the vertical direction & CrossAxisAligment in the horizontal direction.
Container(
color: Colors.grey[300],
width: double.infinity,
height: double.infinity,
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
height: 50,
width: 50,
color: Colors.red,
),
Container(
height: 50,
width: 50,
color: Colors.green,
),
Container(
height: 50,
width: 50,
color: Colors.blue,
),
],
),
),
Column Example 1
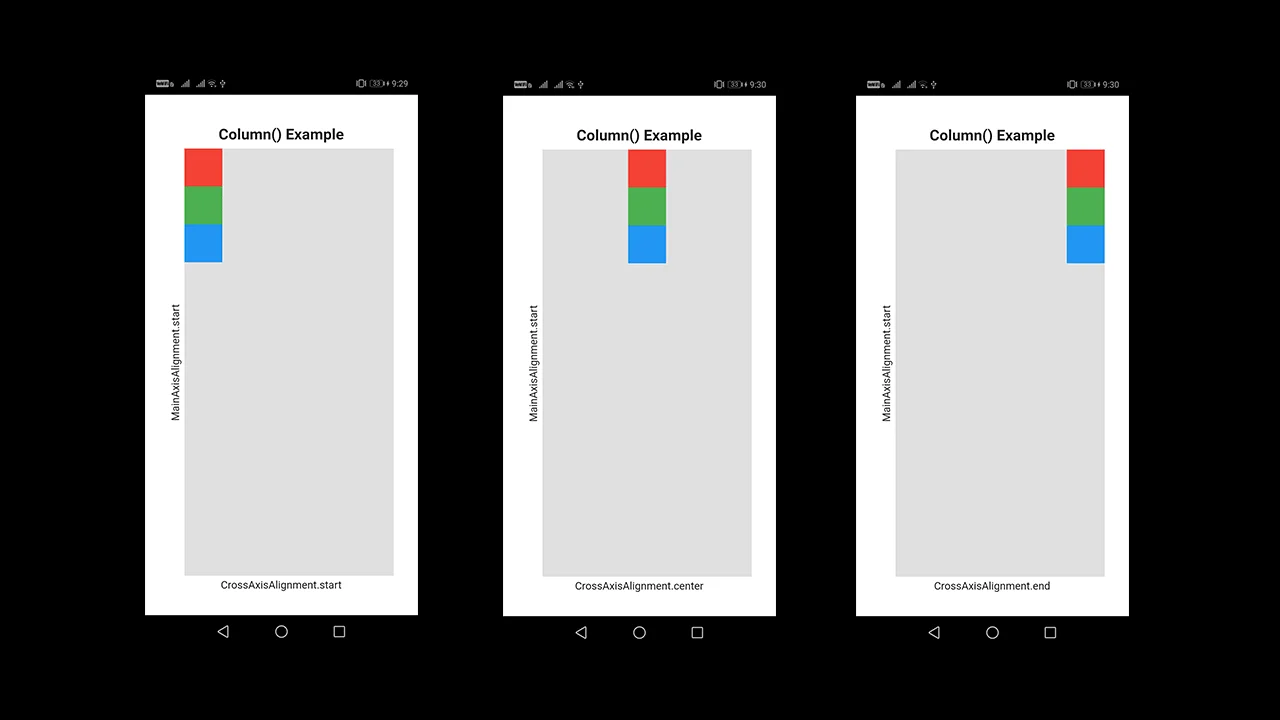
In the above example, we Fixed MainAxisAligment in the start position and changed CrossAxisAligment as a start, center, and end position.
Flutter Column Example 2
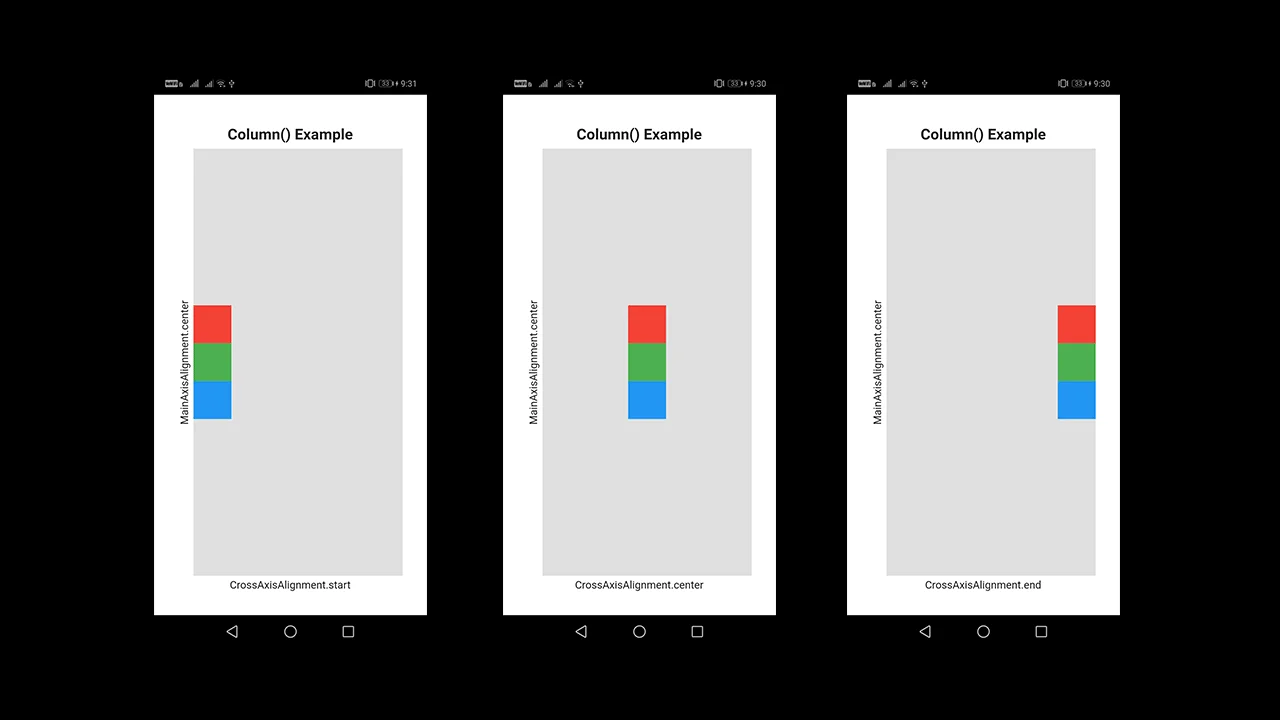
In the above example, we Fixed MainAxisAligment in the center position and changed CrossAxisAligment as a start, center, and end position.
Flutter Column Example 3
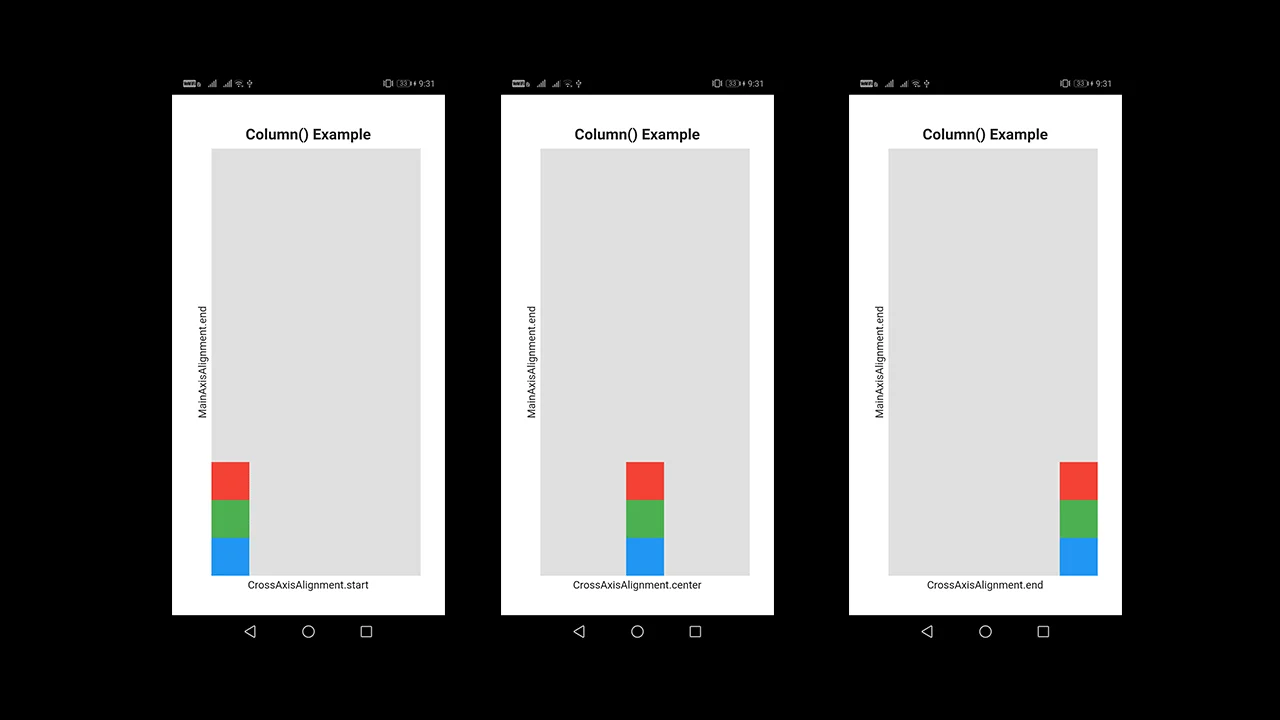
In the above example, we Fixed MainAxisAligment in the end position and changed CrossAxisAligment as a start, center, and end position.
Flutter Column Example 4
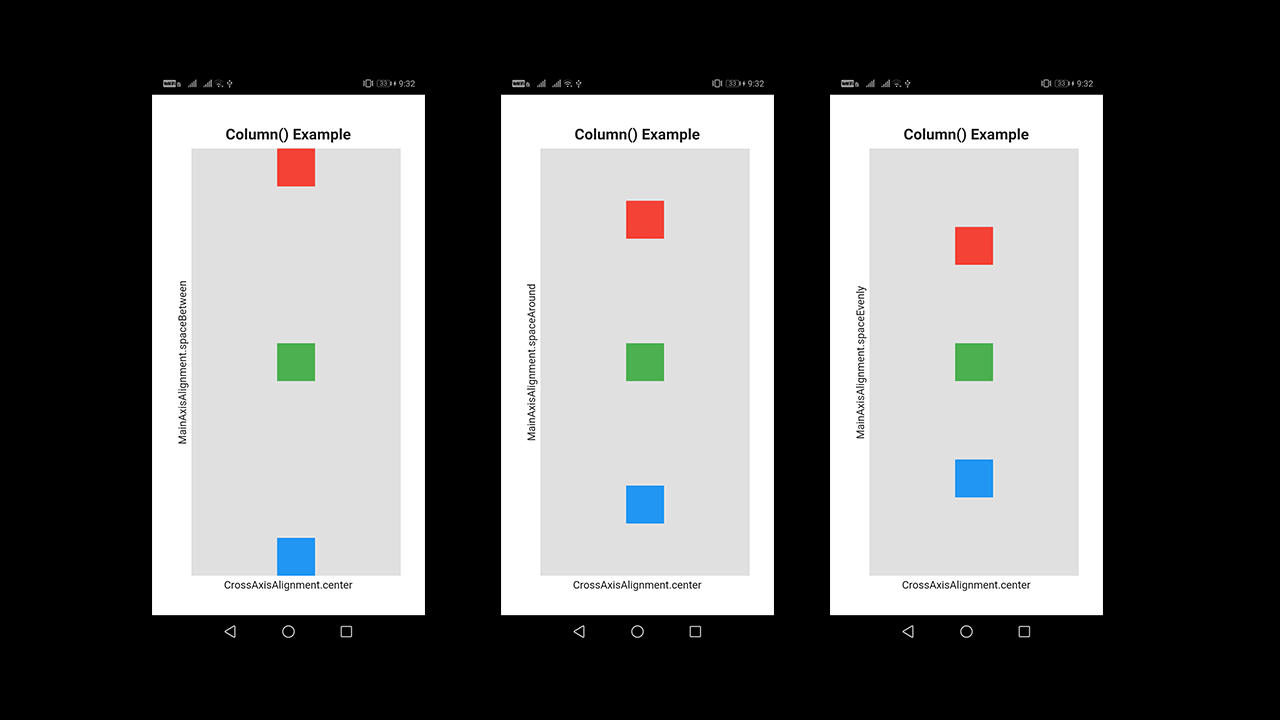
In the above example, we Fixed CrossAxisAligment in the center position and changed MainAxisAligment.
MainAxisAlignment.spaceBetween: First & Last widget no space & space between inner widgets
MainAxisAlignment.spaceAround: All widgets wrapped with some space around.
MainAxisAlignment.spaceEvenly: All widgets wrapped with equal space
Column Example 5
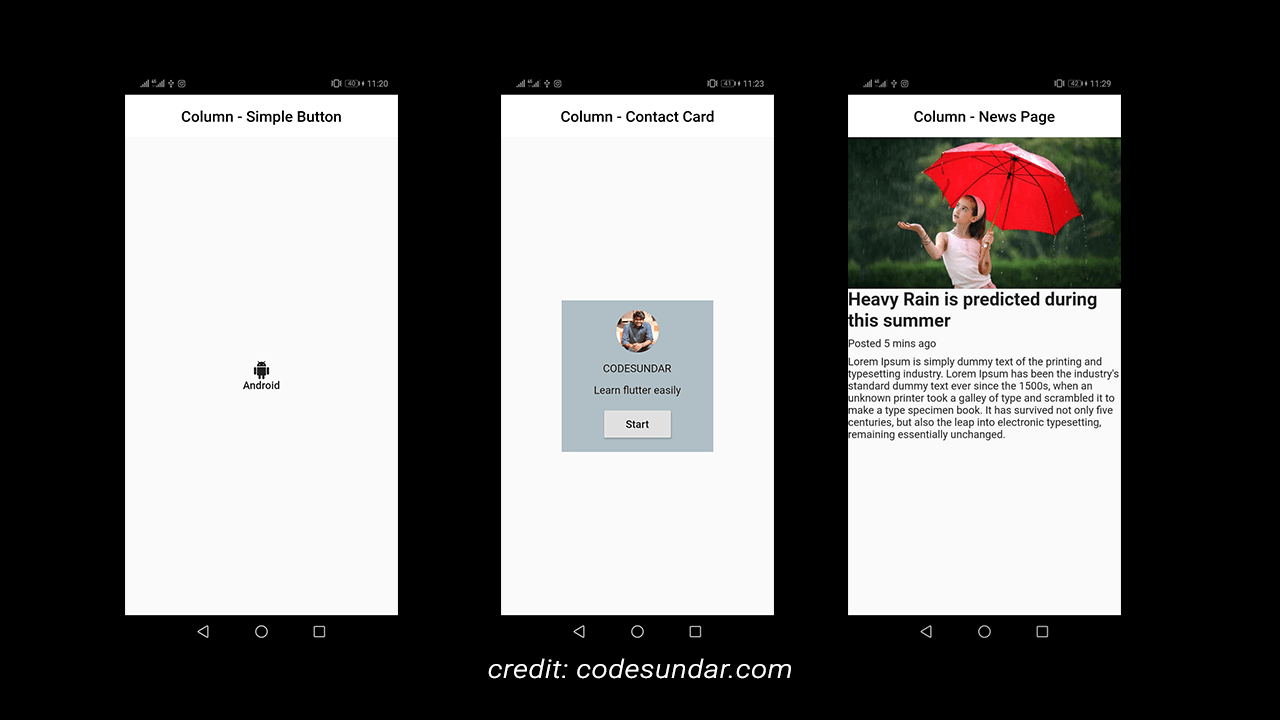
Exploring Row Widget
As said before, the row widget aligns children widget horizontal direction. To change the UI, we need to play with CrossAxisAligment & MainAxisAlignment. In the Row widget, MainAxisAligment will be in the horizontal direction & CrossAxisAligment in the vertical direction.
Container(
color: Colors.grey[300],
width: double.infinity,
height: double.infinity,
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
height: 50,
width: 50,
color: Colors.red,
),
Container(
height: 50,
width: 50,
color: Colors.green,
),
Container(
height: 50,
width: 50,
color: Colors.blue,
),
],
),
),
Flutter Row Example 1
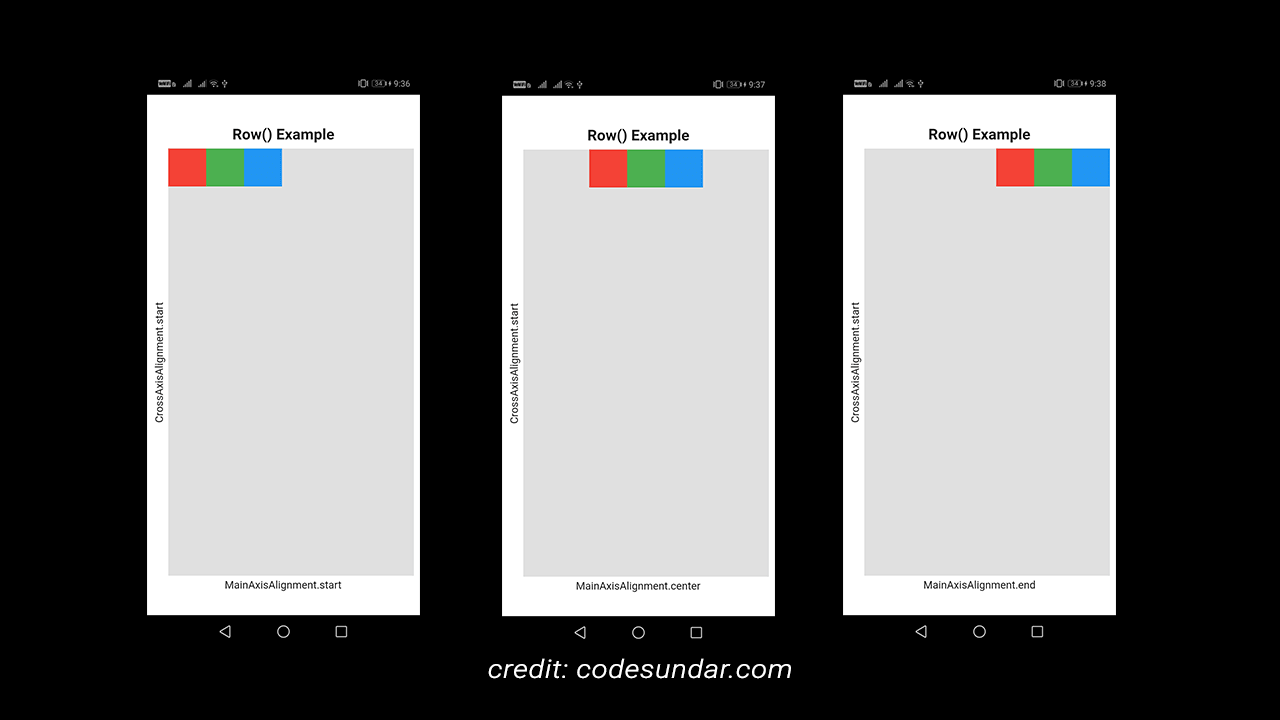
In the above example, we Fixed CrossAxisAligment in the start position and changed MainAxisAligment as a start, center, and end position.
Flutter Row Example 2
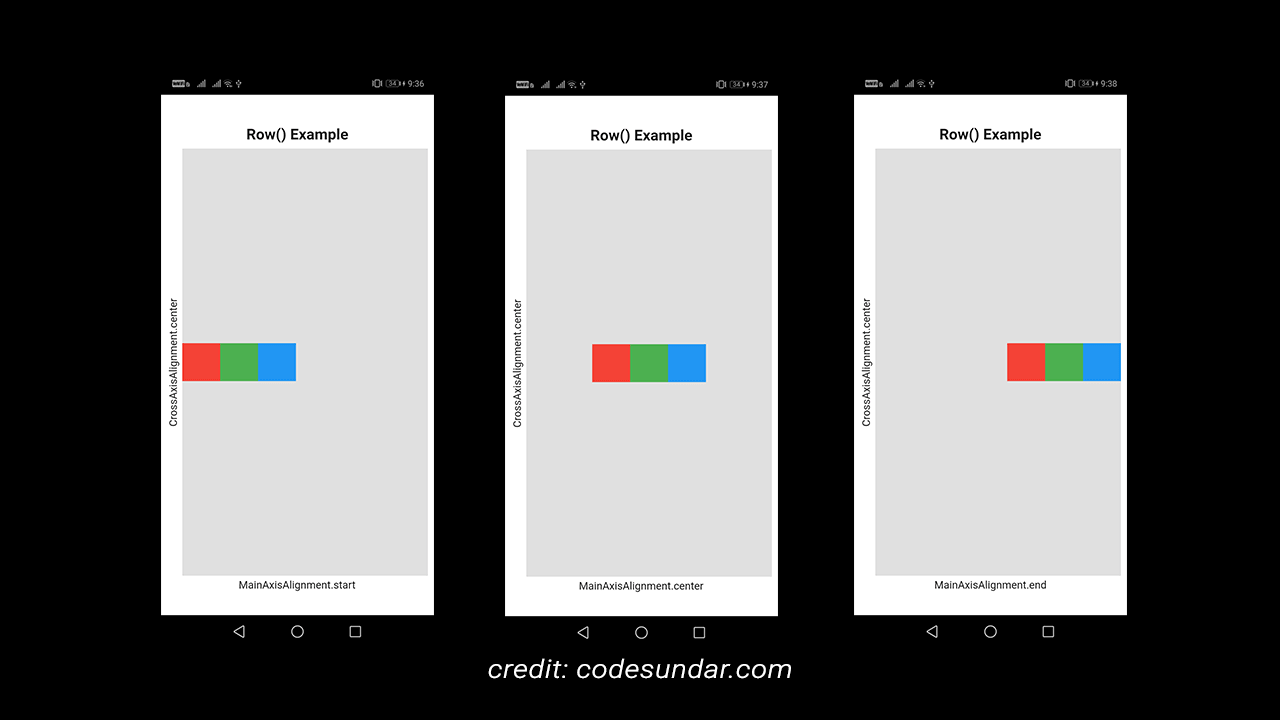
In the above example, we Fixed CrossAxisAligment in the center position and changed MainAxisAligment as a start, center, and end position.
Flutter Row Example 3
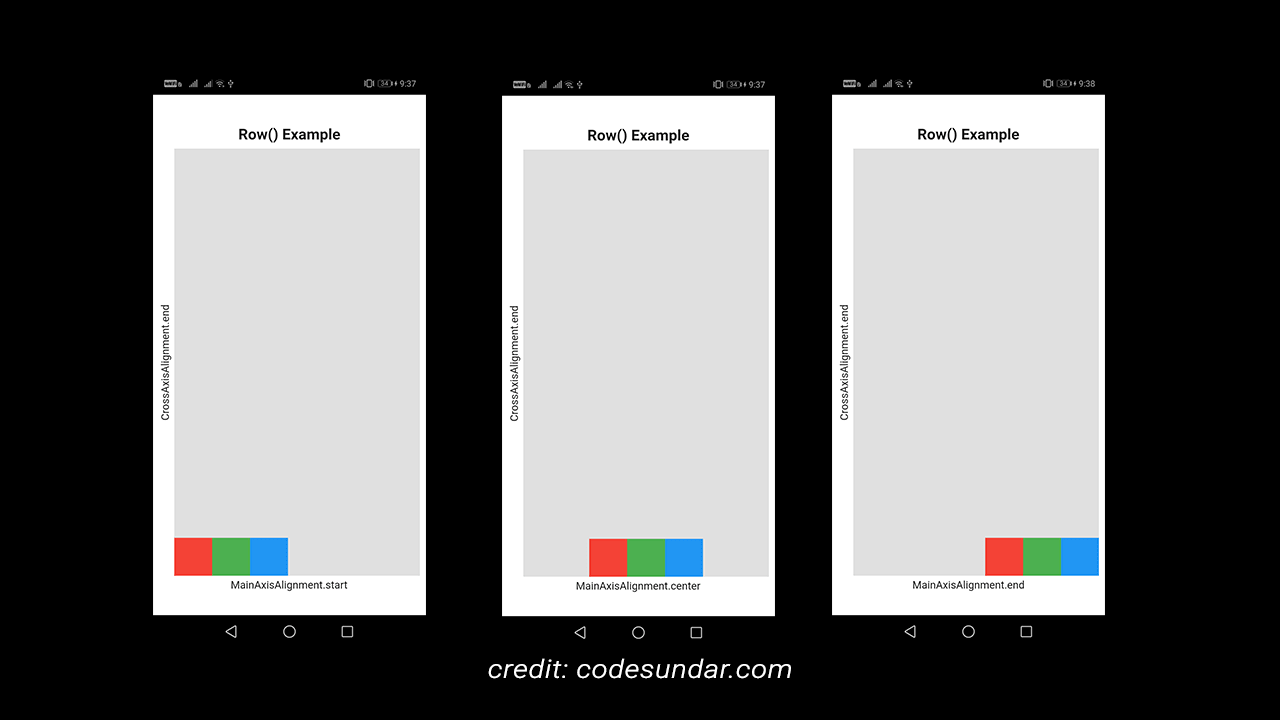
In the above example, we Fixed CrossAxisAligment in the end position and changed MainAxisAligment as a start, center, and end position.
Flutter Row Example 4

In the above example, we Fixed CrossAxisAligment in the center position and changed MainAxisAligment.
MainAxisAlignment.spaceBetween: First & Last widget no space & space between inner widgets
MainAxisAlignment.spaceAround: All widgets wrapped with some space around.
MainAxisAlignment.spaceEvenly: All widgets wrapped with equal space
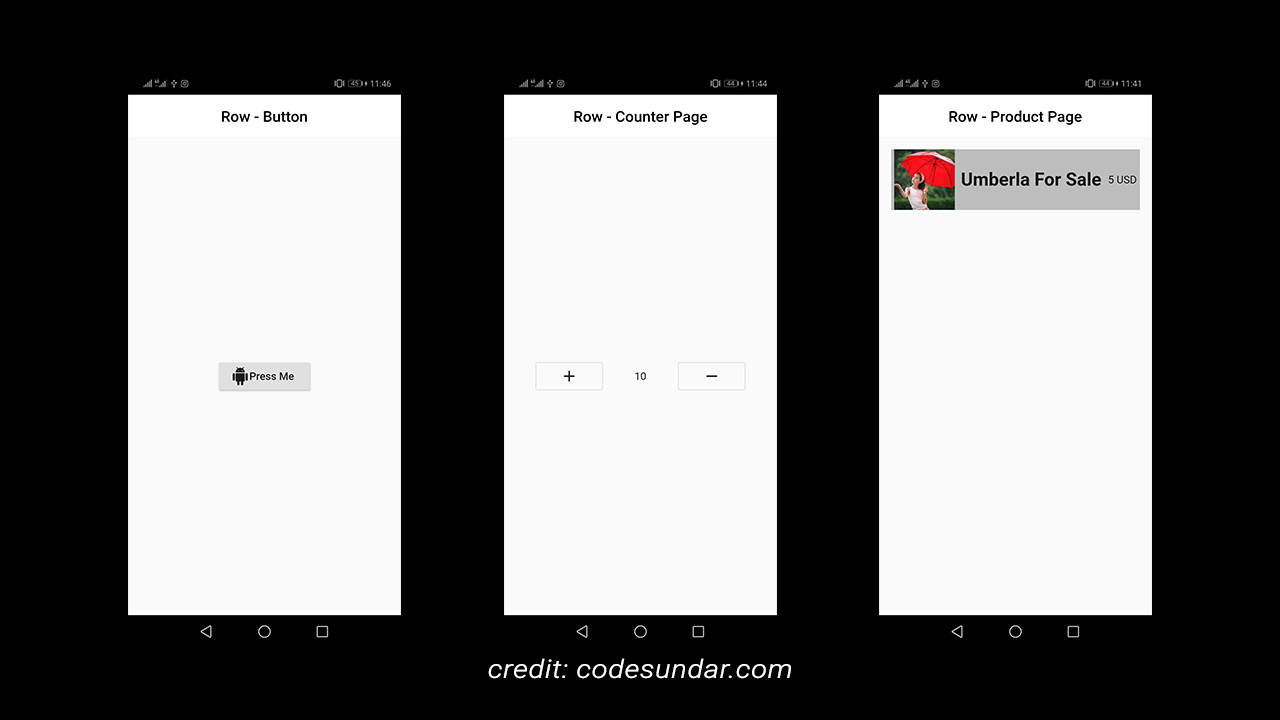
Rows & Column Together
Yes, you can merge row & column together to build complex UI.
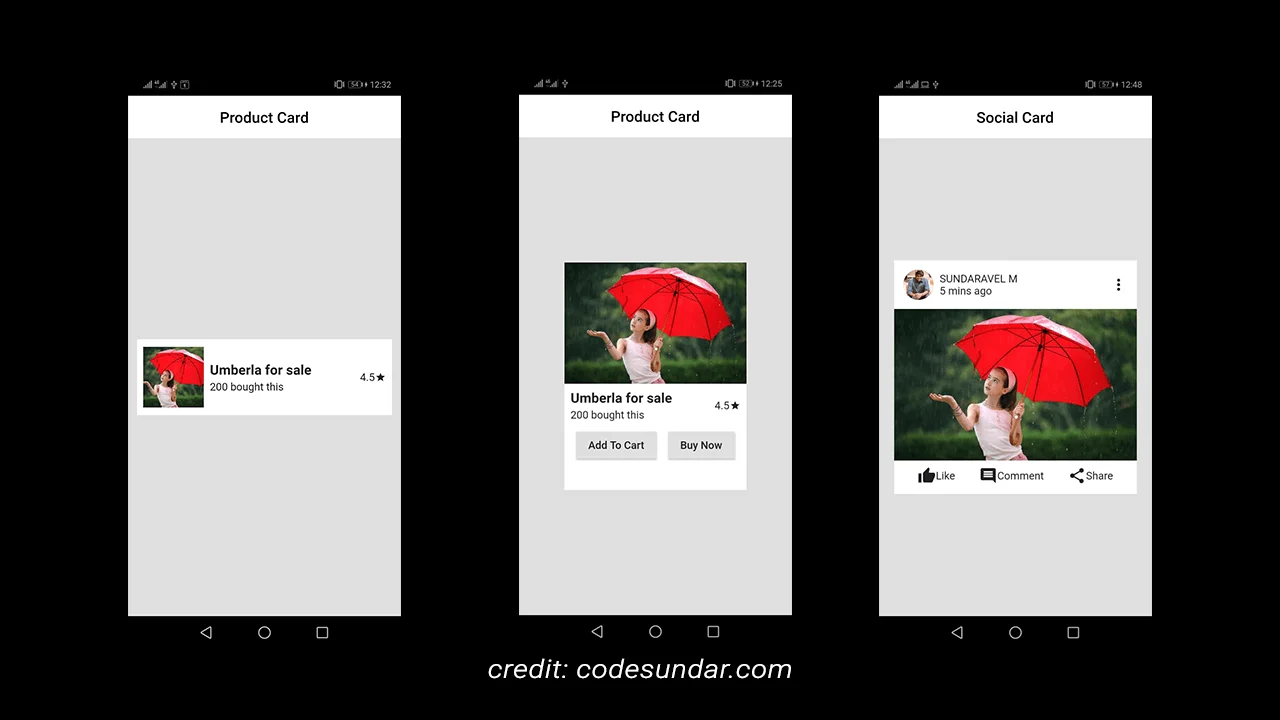
Additional References: