As a part of our flutter tutorial series, we’re going to learn how to work with the flutter share package. Using this, we can share text, images, files from the flutter app to another app. For this example, we’re going to use a package called share
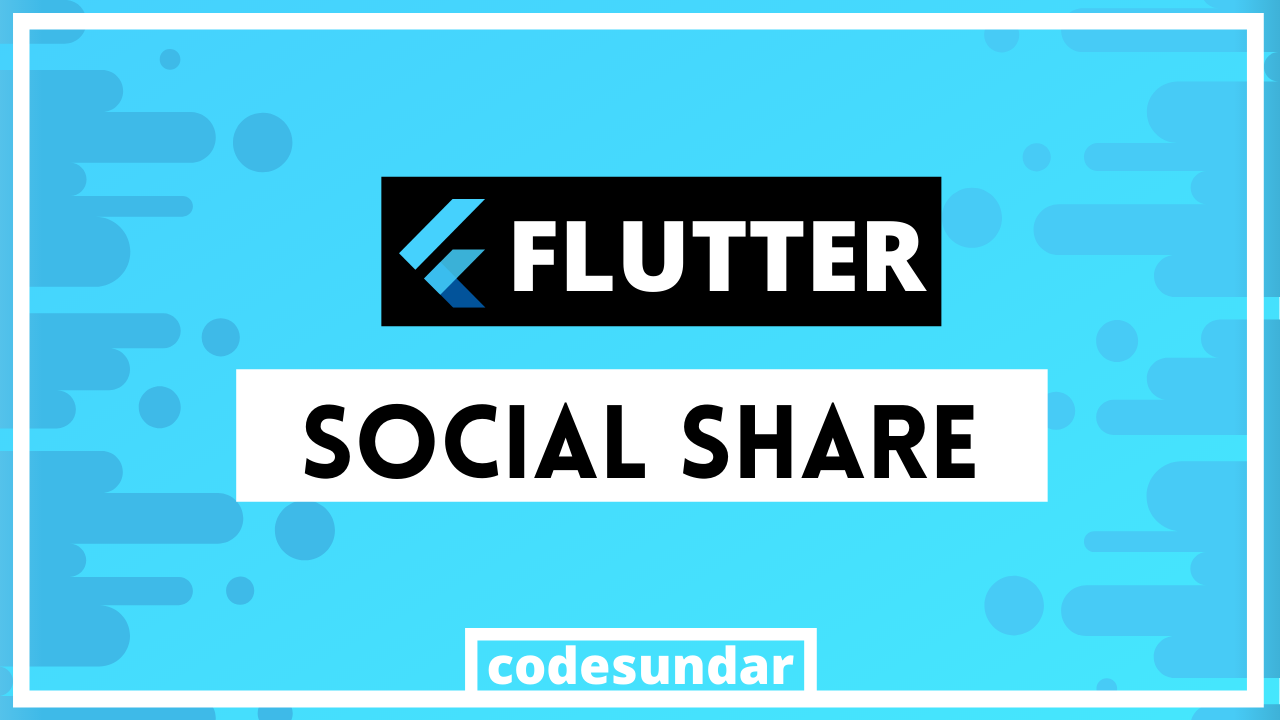
Usage
- Helps you to share text, files to another app
Getting started
To start, we need to create a new flutter project or we can use any existing flutter project.
flutter create learnflutter
cd learnflutter
To use the share package, we need to add it into pubspec.yaml
dependencies:
share: ^0.6.5+4
After the plugin is added we need to run flutter pub get
to install the required dependencies
Sharing Text with Flutter
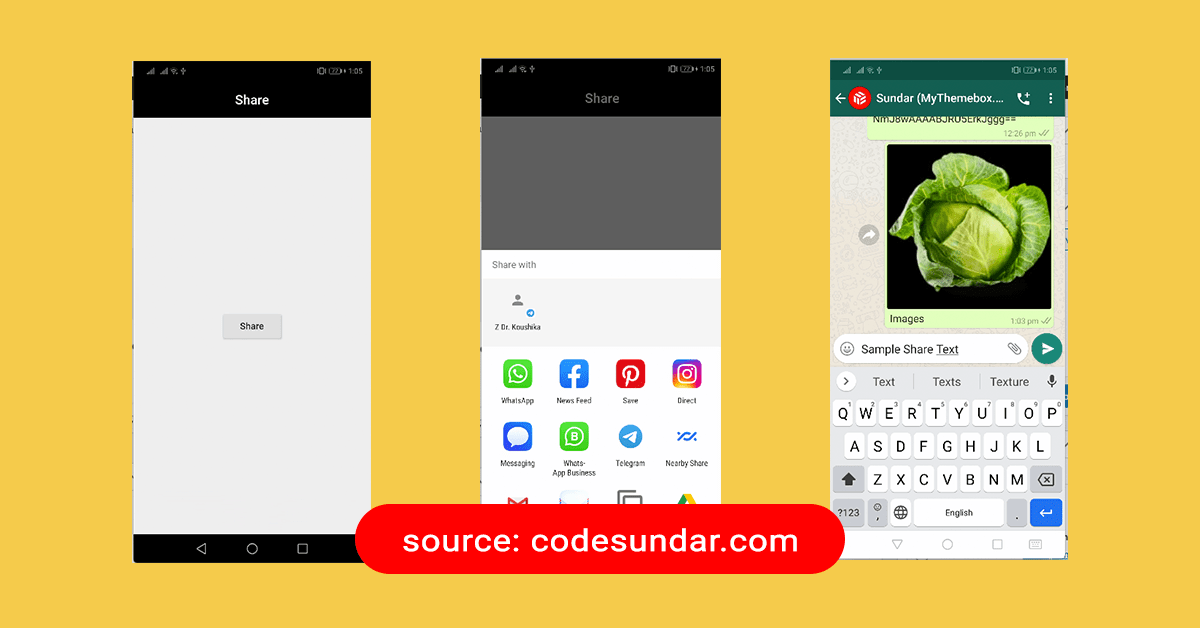
import 'package:flutter/material.dart';
import 'package:share/share.dart';
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Share"),
),
body: Center(
child: RaisedButton(
child: Text("Share"),
onPressed: () {
Share.share("Sample Share Text");
},
)),
);
}
}
Explanation
- As a first step, we imported the share plugin
- When the user pressed the button, we called
Share.share()
to share custom text
How to share files in flutter?
In case, if you want to share files we can use Share.shareFiles()
. which required a directory path to share. For that, we’re going to use a package called file_picker
Add this into your pubspec.yaml
& run flutter pub get
dependencies:
file_picker: ^2.0.13
If you faced any issue while building the app, please refer to troubleshooting: http://github.com/miguelpruivo/flutter_file_picker/wiki/Troubleshooting
Sharing Single File in Flutter
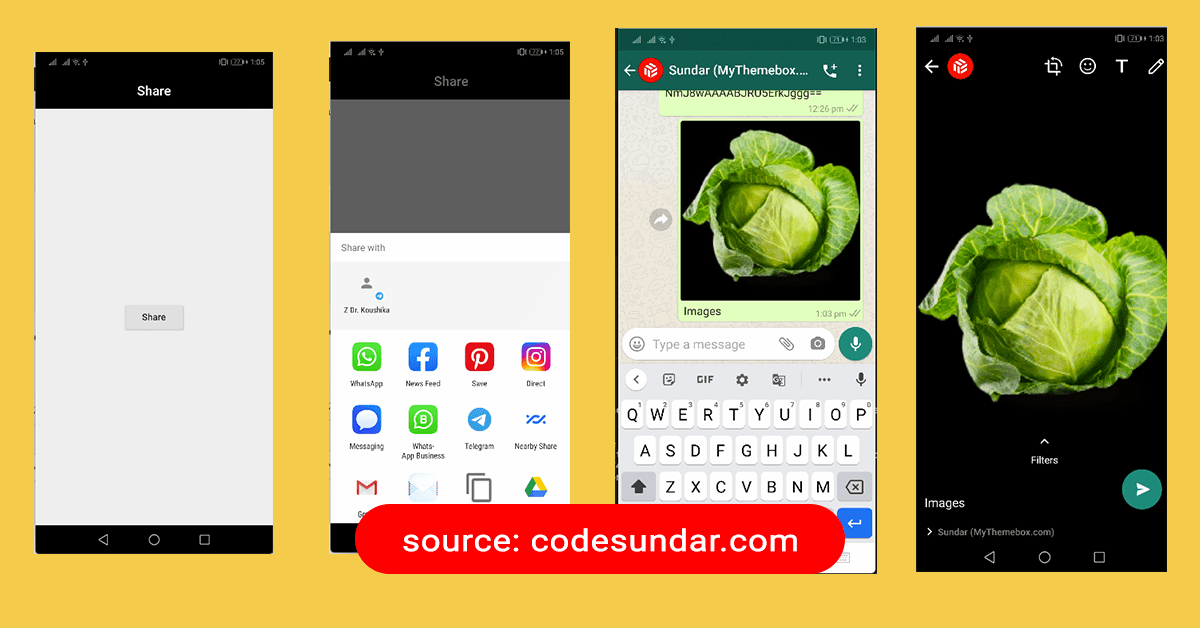
RaisedButton(
child: Text("Share"),
onPressed: () async {
FilePickerResult result = await FilePicker.platform.pickFiles();
if (result != null) {
print(result.files.single.path);
Share.shareFiles(["${result.files.single.path}"], text: "Images");
}
},
),
In the above example, we get only one file path and passing into Share.shareFiles
Sharing Multiple Files in Flutter
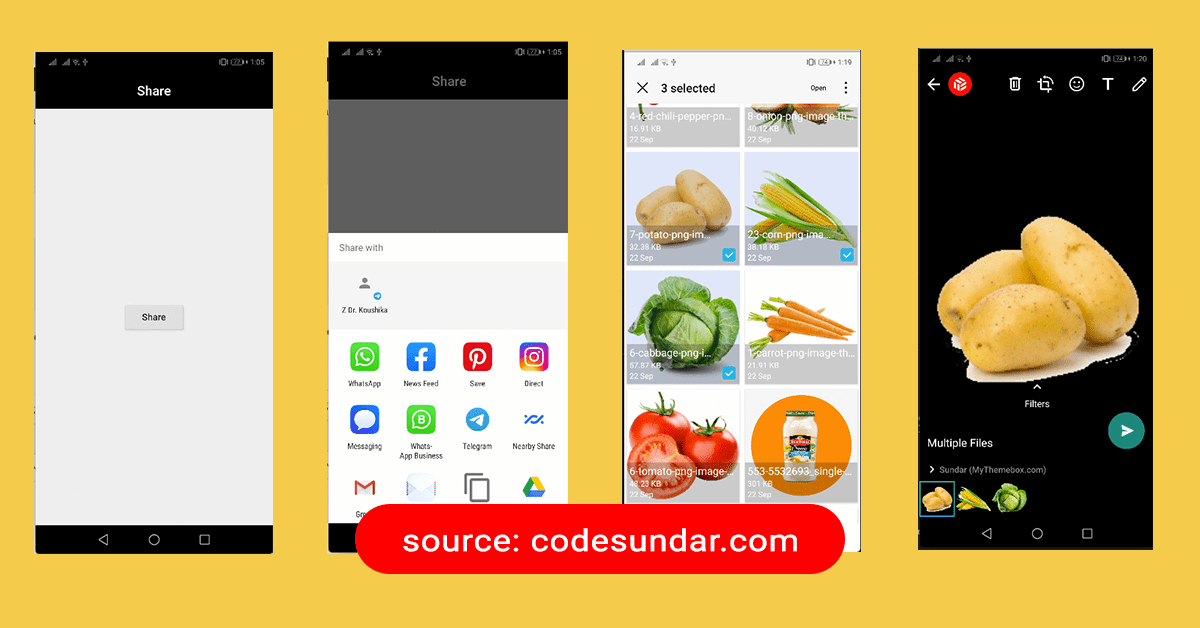
RaisedButton(
child: Text("Share"),
onPressed: () async {
FilePickerResult result =
await FilePicker.platform.pickFiles(allowMultiple: true);
if (result != null) {
List files = result.paths.map((f) => File(f).path).toList();
Share.shareFiles(files, text: "Multiple Files");
}
},
),
In the above example, we’re fetching multiple files and convert into a List of files path and then pass to the share function
How to Restrict File Extension
RaisedButton(
child: Text("Share"),
onPressed: () async {
FilePickerResult result = await FilePicker.platform.pickFiles(
allowMultiple: true,
allowedExtensions: ['jpg', 'pdf'],
type: FileType.custom,
);
if (result != null) {
List files = result.paths.map((f) => File(f).path).toList();
Share.shareFiles(files, text: "Multiple Files");
}
},
),
Here, we restricted file extension using allowedExtensions
& FileType.custom